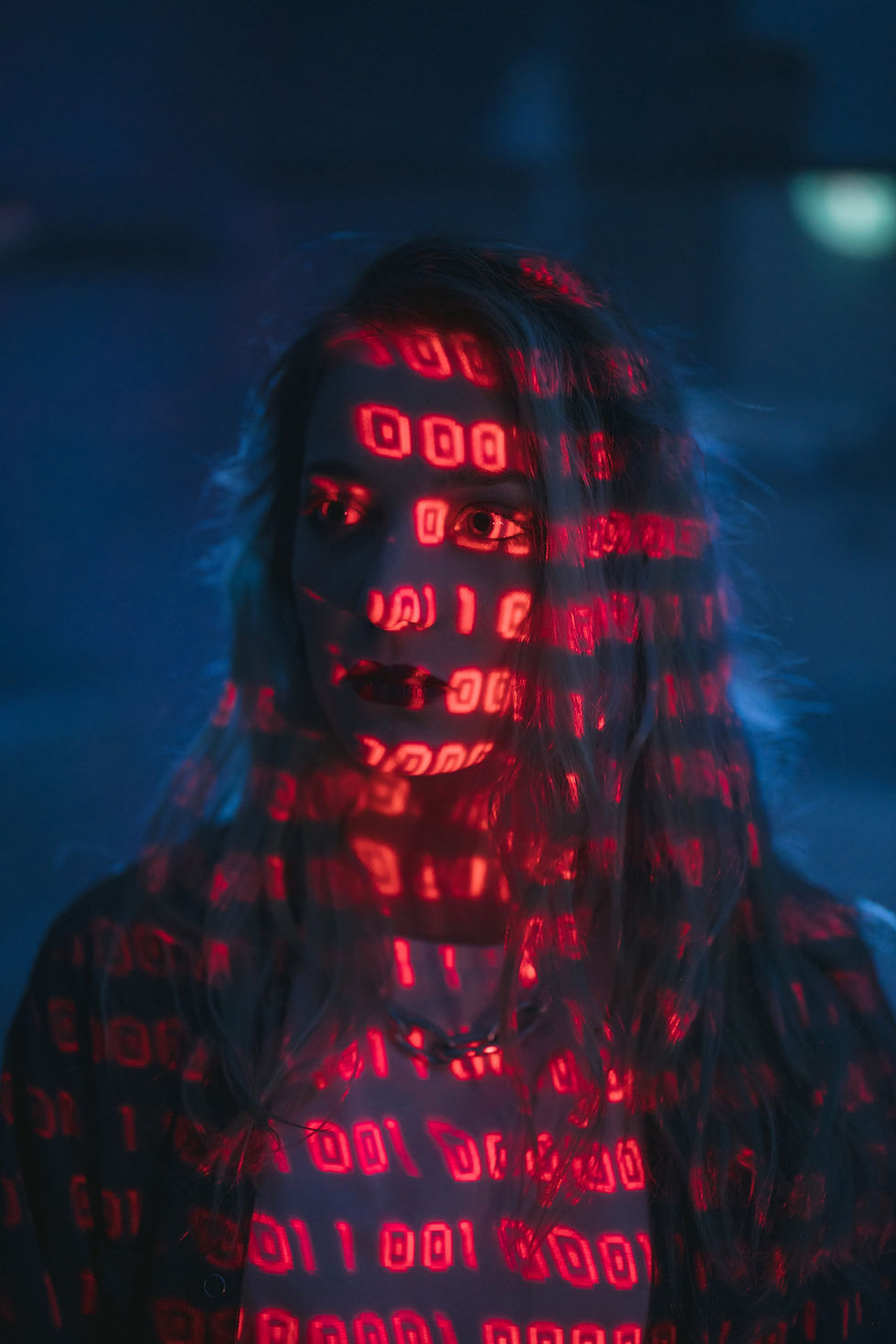
While fundamental programming knowledge like loops and arrays is essential, mastering design patterns elevates your code beyond basic functionality. Design patterns provide reusable solutions to common programming problems, offering several benefits:
1. Readability: Well-known design patterns make your code easier to understand for yourself and others. By following established patterns, code becomes more consistent and predictable, reducing the learning curve for anyone reviewing or modifying it. This is especially crucial in collaborative projects or when revisiting your own code after a long period.
2. Maintainability: Code written with design patterns is often more modular. This means it's broken down into smaller, independent units (classes, functions) with well-defined responsibilities. This modularity makes the code easier to modify or extend in the future. For example, if you need to add a new feature, you can focus on modifying a specific module without affecting unrelated parts of the code.
3. Reusability: Many design patterns are not limited to a single specific problem. Once you understand and implement a pattern, you can often apply it in different contexts to solve similar problems. This saves time and effort in development, allowing you to focus on new challenges rather than reinventing the wheel for every task.
4. Efficiency: Some design patterns can optimize code performance. For example, the "Singleton" pattern, which ensures only one instance of a class exists, can be useful for managing resources efficiently. However, it's important to choose the right pattern for the specific situation, as applying patterns unnecessarily can sometimes add complexity without offering significant performance gains.
This article provides a brief overview of several core design patterns that are commonly used in various programming languages:
Singleton: This pattern ensures that only one instance of a specific class exists throughout the application. It's often used for managing global resources or configuration settings. A good example is a "Logger" class that handles all logging activities in a program – you only need one instance of this class to avoid duplicate logs and maintain consistency.
Factory: This pattern allows you to create objects without exposing the specific creation logic. Instead of directly instantiating objects, you use a factory method or class that takes necessary parameters and returns the appropriate object type. This promotes flexibility and decoupling, as the code that consumes the object doesn't need to know the exact implementation details of its creation.
Observer: This pattern defines a one-to-many dependency relationship between objects. When a subject object (observable) changes its state, it notifies all its dependent objects (observers) about the change. This pattern is useful for building loosely coupled systems where one object's changes can trigger actions in other parts of the application. An example could be a stock price ticker that notifies multiple listeners (observers) about price updates.
Decorator: This pattern allows you to dynamically add new functionalities to an existing object without modifying its original code. It's achieved by wrapping the original object with a decorator object that provides additional functionality. Decorators are widely used in various languages for functionalities like logging, caching, or security checks.
Learning and applying design patterns takes practice, but it can significantly improve your coding skills and the overall quality of your code. By understanding the benefits and various types of design patterns, you can choose the appropriate ones to make your code cleaner, more maintainable, reusable, and potentially more efficient
Comments